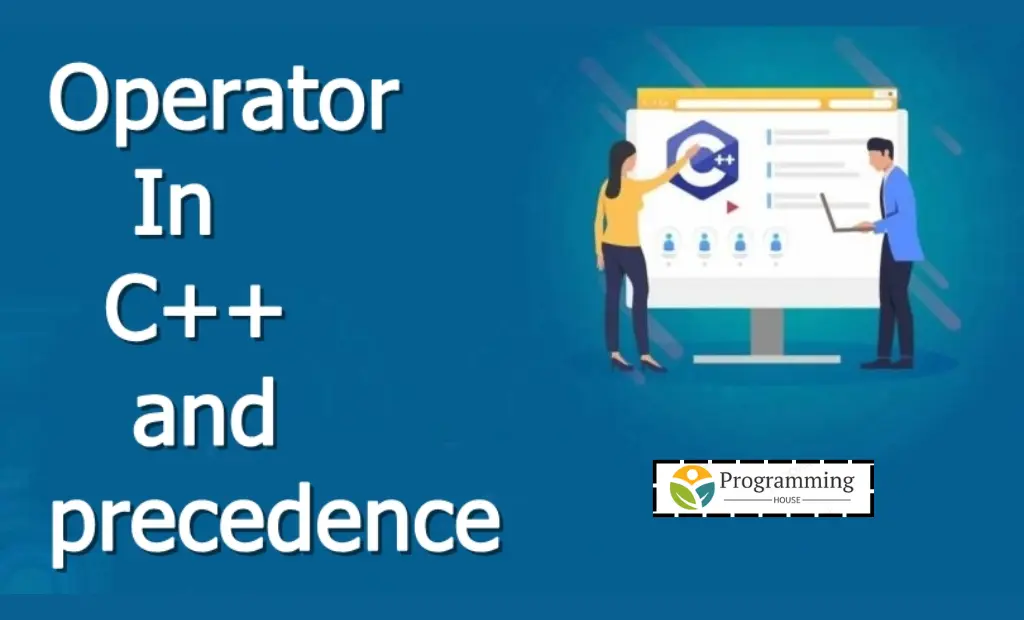
Key Highlights
- Operators in C++ symbols that perform specific or mathematical functions on operands.
- There are several types operators in C++, including arithmetic operators, relational operators, logical operators, assignment operators, bitwise operators, and special operators.
- Operators in C++ are essential for performing various like addition, subtraction, multiplication,, comparison, and more.
- Understanding the precedence and associativity of operators is crucial in determining the order of evaluation in complex expressions.
- Common mistakes with operators in C++ include using the wrong operator, incorrect usage of assignment operators, and not considering operator precedence.
- Knowing how operators work in C++ is crucial for writing efficient and error-free code.
Introduction
Operators in C++ are symbols that help programmers do logical or math tasks on operands. These symbols are crucial for operations in a program. C++ is a popular language with many operators for manipulating data, like addition or comparison. The operators are split into categories based on what they do, such as arithmetic or logical. Each type serves a specific function for different tasks. Knowing how they work is vital for writing error-free code efficiently. Understanding their order of evaluation by precedence and associativity is key in complex expressions. By using them correctly, programmers can process data to fit their program’s needs well. In the upcoming sections, we will cover the basics and types of C++ operators in detail, discussing their importance and common mistakes to avoid.
Understanding the Basics of Operators in C++
In C++, operators are symbols for logical or mathematical functions on operands. They are crucial building blocks for programming. Different types of operators in C++ include arithmetic, relational, logical, assignment, bitwise, and special operators. Each type has a unique purpose for various operations. Using operators correctly helps programmers control and process data effectively. Understanding these basics is vital for writing efficient code without errors.
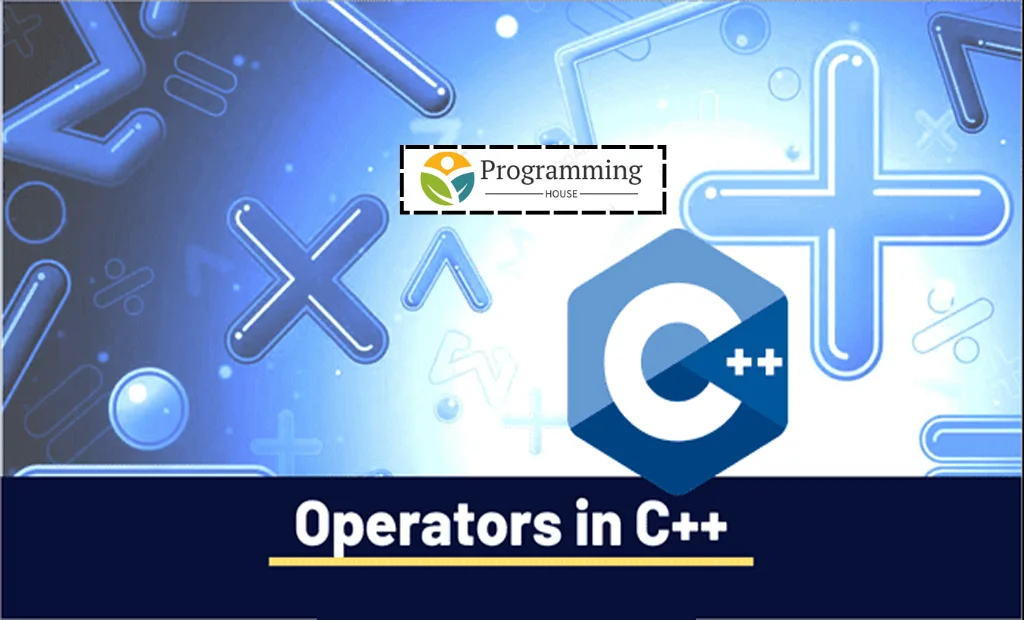
Definition and Importance of Operators
Operators in C++ are symbols that do specific functions on data. They come in two types: unary and binary. Unary ones work on one piece of data, like adding or subtracting. Binary ones work on two pieces, doing tasks like adding or dividing.
Unary operators change values, while binary operators do math operations. These symbols help programmers perform calculations and comparisons efficiently. In programming languages such as C++, they are essential for writing effective code. Without them, language capabilities would be restricted, making coding difficult.
Categories of Operators in C++
There are different groups of operators in C++. They serve specific purposes. Some categories are:
- Math Operators: Perform basic math like addition, subtraction, multiplication, division, and modulus.
- Compare Operators: Compare values to find relationships like equal, not equal, greater than, or less than.
- Logic Operators: Combine conditions using AND, OR, and NOT.
- Set Values: Assign values to variables.
- Bit Operators: Work on individual bits of data.
- Special Operators: Include ternary, comma, and sizeof.
Knowing these operator categories is crucial for writing good code efficiently in C++.
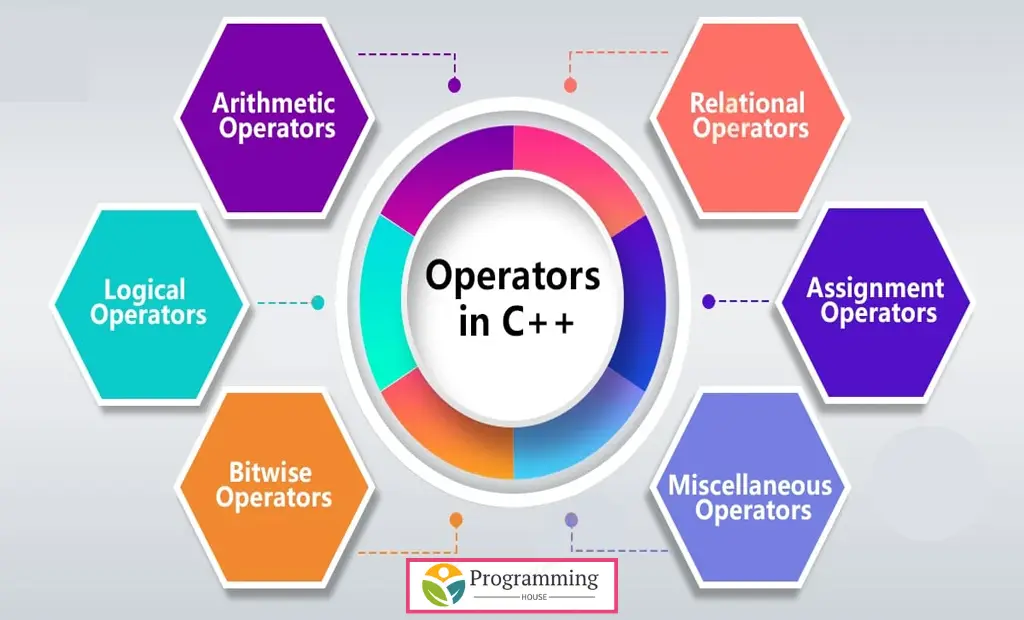
Arithmetic Operators Explored
Arithmetic operators in C++ do basic math on operands. They include addition, subtraction, multiplication, division, and modulus.
The addition operator adds two operands to get their sum. The subtraction operator subtracts the second operand from the first to find the difference.
The multiplication operator multiplies two operands to get their product. The division operator divides the first operand by the second to get the quotient.
The modulus operator divides the first operand by the second to find the remainder. These operators are vital for math in C++.
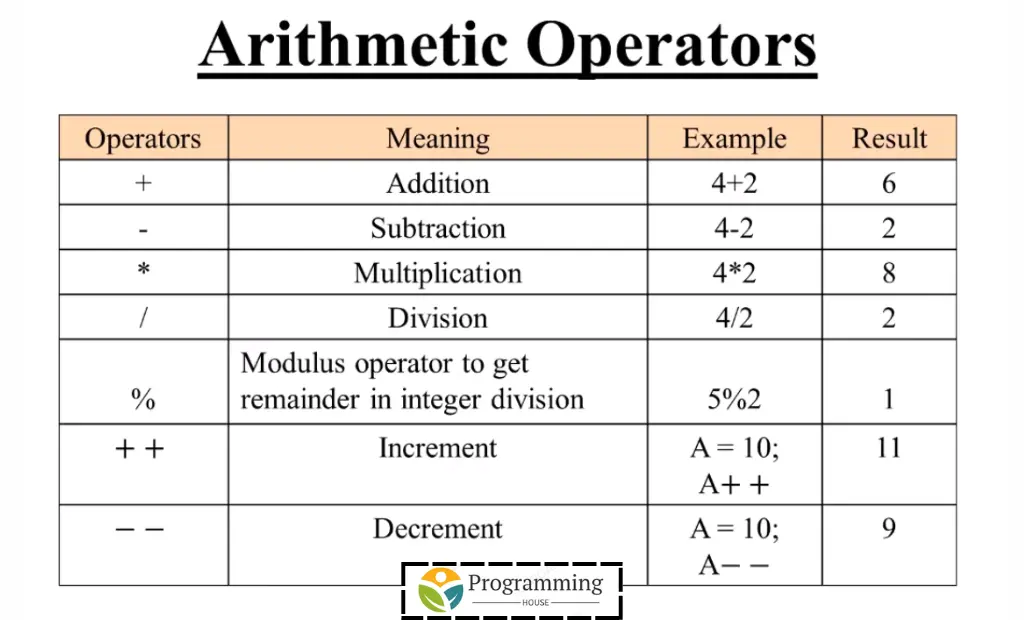
Addition, Subtraction, Multiplication, and Division
The plus sign (+) in C++ adds two numbers to find the sum like “a + b”.
The minus sign (-) in C++ subtracts one number from another to give the difference as “a – b”.
The asterisk (*) in C++ multiplies two numbers to get the product as “a * b”.
The forward slash (/) in C++ divides one number by another to provide the quotient as “a / b”.
These math symbols are vital for calculations in C++.
Modulus Operator and Its Use Cases
The modulus operator (%) in C++ divides numbers and finds the remainder. It works only with whole numbers.
It helps determine if a number is even or odd. If dividing by 2 leaves no remainder, it’s even; otherwise, it’s odd.
One more use is getting the last digit of a number. Modulo 10 gives that last digit.
It can also help cycle through a set range. For hours, modulo 24 keeps values from 0 to 23.
In C++, the modulus operator is handy for many calculations.
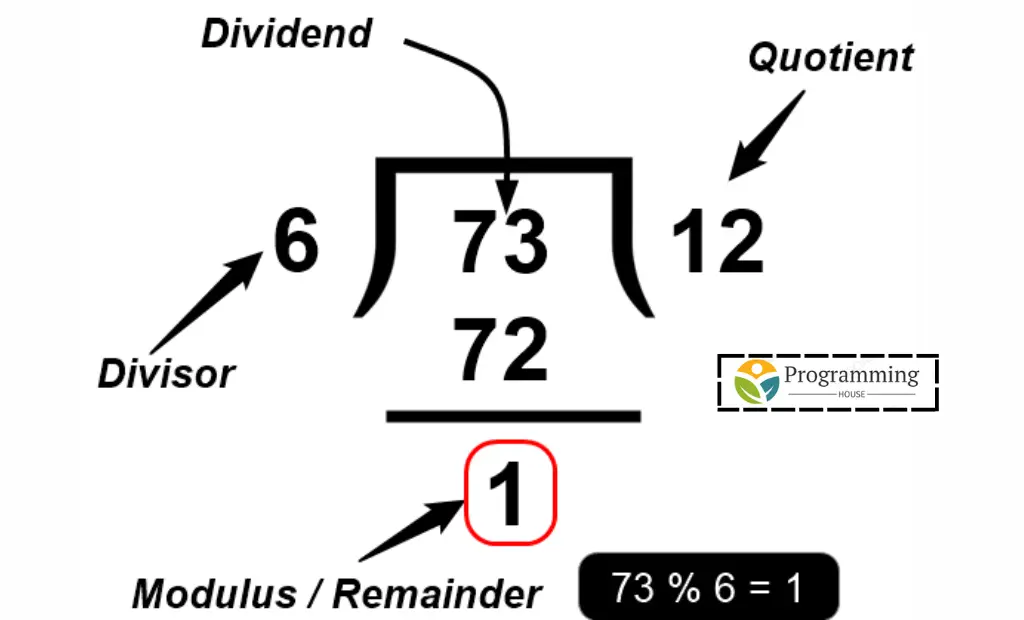
Relational Operators
Relational operators in C++ compare values. They show if values are equal, not equal, greater, or less. Operators include ==, !=, >, >=, <, and <=.
These operators give true (1) or false (0) results. “a > b” is true if a is more than b; otherwise false.
Use them in decisions within loops and conditional statements widely based on the comparison outcomes.
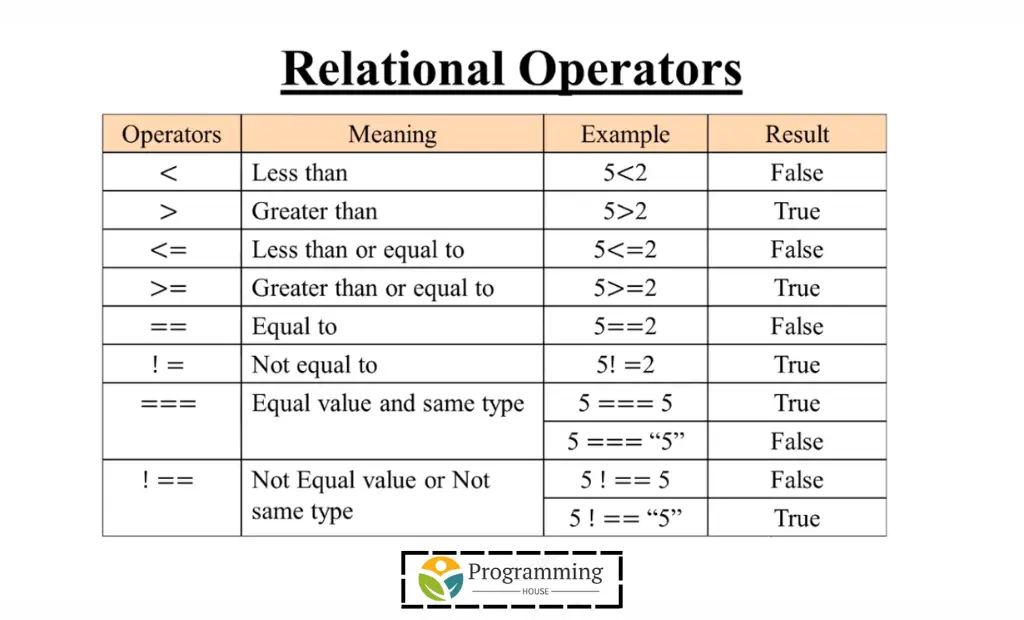
Understanding Equality and Inequality Operators
The equality operator (==) in C++ compares two operands to check if they are the same. It returns true and otherwise, it returns false. For instance, “a == b” is true if a equals b; otherwise, it’s false.
The inequality operator (!=) in C++ compares two operands to check if they are different. It returns true if they’re not equal; otherwise, it returns false. For example, “a != b” is true if a doesn’t equal b; otherwise, it’s false.
These operators are vital for comparing values in C++.
Greater Than and Less Than Operators
The “>” in C++ compares values to check if the first is greater than the second. It returns true if so; otherwise, false. For instance, “a > b” is true when a is greater than b.
The “<” in C++ compares values to check if the first is less than the second. It returns true if so; otherwise, false. For example, “a < b” is true when a is less than b.
These operators are widely used for comparisons in C++ loops and conditional statements.
Logical Operators
Logical operators in C++ help with combining conditions to create a single boolean expression. They include AND (&&), OR (||), and NOT (!). These operators are crucial for controlling program flow based on specific criteria. They are commonly used in if-else statements and loops to make programming more flexible and efficient. Knowing how to use them well is vital for writing clean code in C++. Proper use of logical operators ensures that programs execute operations accurately based on given conditions.
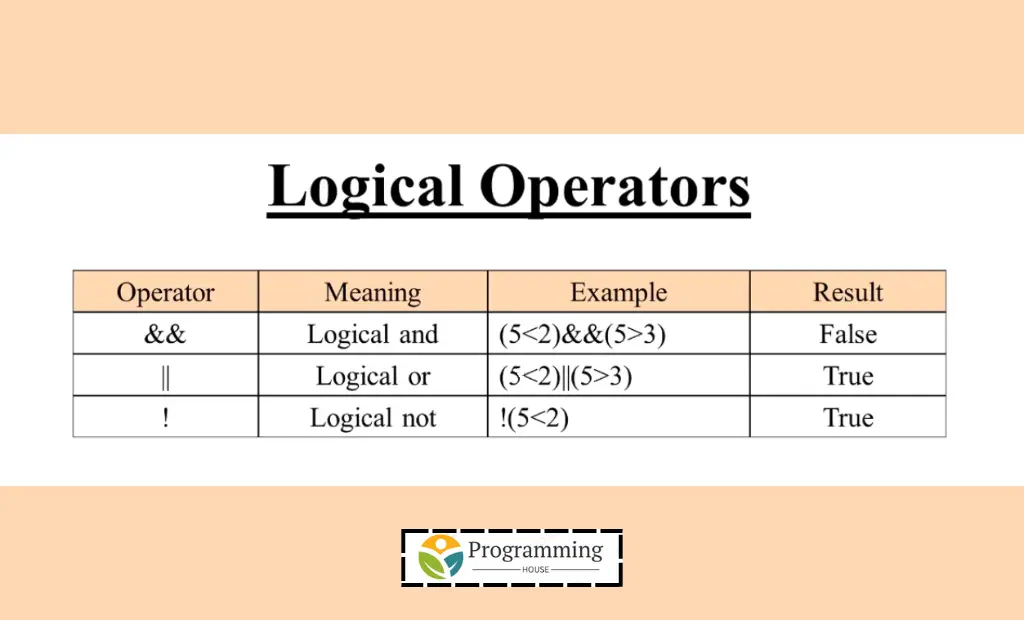
The Role of AND, OR, and NOT Operators
In C++, simple operators like AND, OR, and NOT are essential for checking true or false statements. The AND operator (&&) needs both parts to be true for an overall truth. On the contrary, the OR operator (||) only requires one part to be true for a final truth. The NOT operator (!) reverses the truth value of a statement. These operators help in directing program flow based on conditions. Mastering these operators improves logical thinking in C++ programming.
Practical Applications of Logical Operators
Logical operators in C++ are crucial for checking conditions and making decisions based on rules. They help control program flow by creating Boolean expressions. The AND operator (&&) needs both conditions to be true, while the OR operator (||) needs at least one. These tools are vital when dealing with complex conditions. Programmers use them along with other operators to build detailed decision-making processes in their code, ensuring programs work accurately and efficiently.
Increment and Decrement Operators
Increment and decrement operators in C++ are used to increase or decrease a variable by 1. These operators may be prefix or postfix.
The prefix increment operator (++) adds 1 to a variable before using it in an expression, like “++a”.
The postfix increment operator (++) adds 1 to a variable after using it in an expression, like “a++”.
The prefix decrement operator (- -) subtracts 1 from a variable before using it in an expression, like “–a”.
The postfix decrement operator (- -) subtracts 1 from a variable after using it in an expression, like “a–“.
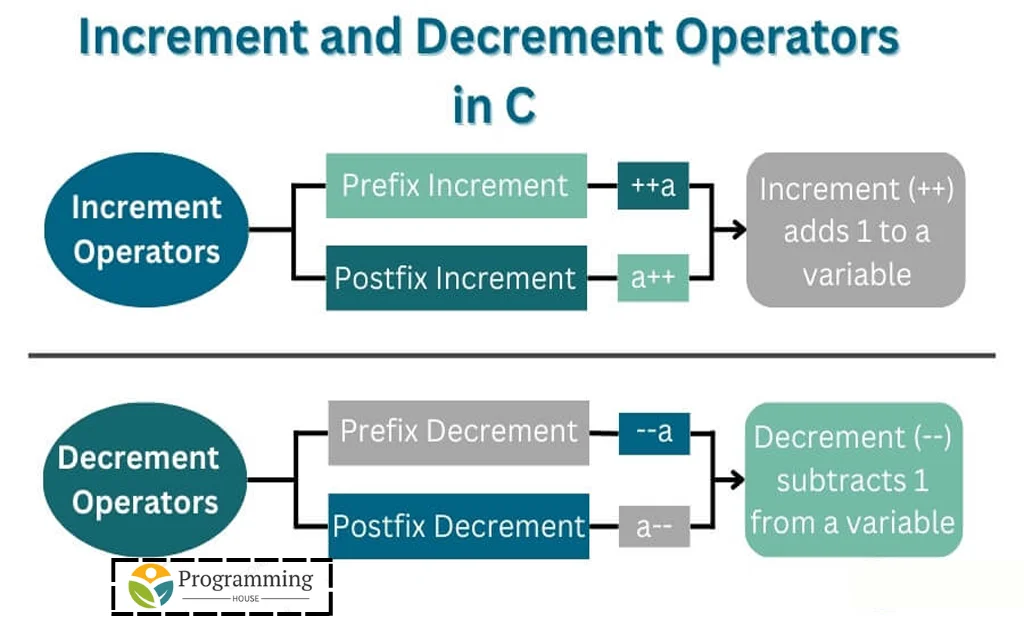
Prefix vs Postfix in C++
In C++, the prefix (++a) and postfix (a++) increment operators behave differently. The prefix operator increments before using the new value, while the postfix operator uses the current value before incrementing it. Understanding this difference is essential in C++ programming.
Scenarios for Using Increment and Decrement Operators
Increment and decrease tools in C++ are often used in many situations. The increment tool (++) can be used to go through a loop, increasing a variable each time. For instance, in a for loop, it can adjust the iteration.
The decrease tool (- -) is handy when a variable needs to be lowered by 1, like in countdowns or reverse loops. These tools also find the original value of a variable before changing it.
For instance, in expressions like “a = ++b” or “a = b++”, the initial b value is assigned to a. Knowing when and how to apply these tools is vital for writing efficient C++ code.
Assignment Operators in Detail
Assignment operators in C++ are used to give values to variables. The basic one is the (=) operator. It adds the value on the right into the variable on the left.
Besides (=), C++ has compound assignment operators. They mix an arithmetic operator with (=). These operators do a math operation and assign the answer to the variable.
Examples of compound assignment operators are += (add and assign), -= (subtract and assign), *= (multiply and assign), /= (divide and assign), and %= (modulus and assign).
Assignment operators are important for changing and updating variable values in C++.
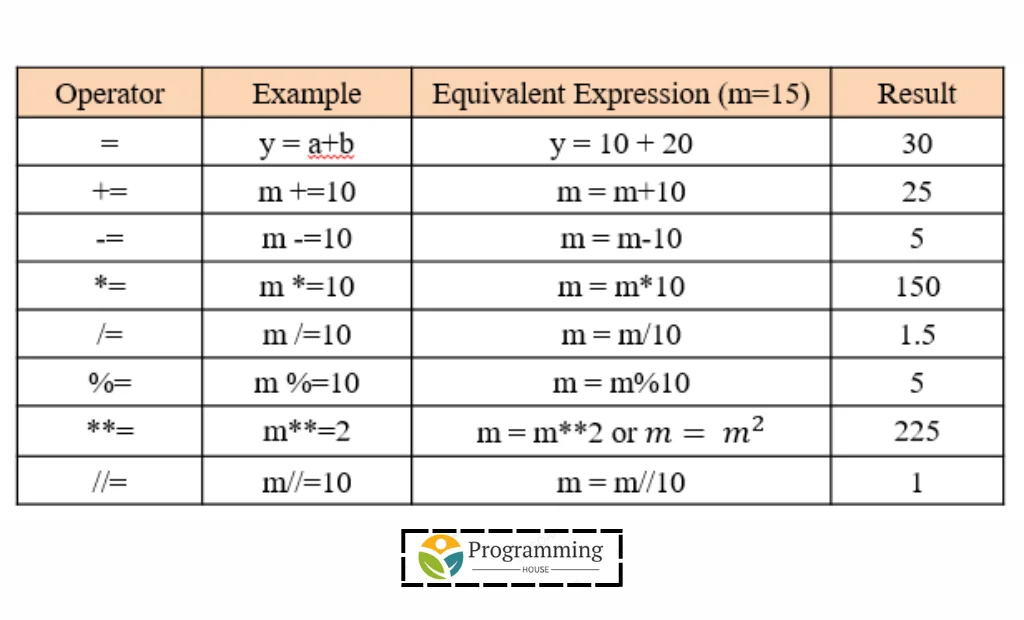
Simple Assignment and Compound Assignment
The basic way to assign values in C++ is using the = operator. It sets the right value to the left variable. For instance, “a = 5” gives a the value of 5.
Compound operators in C++ merge an arithmetic operator with =. They do a math operation and assign the outcome to the variable.
One example is +=, which adds the right value to the left variable and assigns it. So, “a += 5” means “a = a + 5”.
Other compound operators are -= (subtract and assign), *= (multiply and assign), /= (divide and assign), and %= (modulus and assign).
Simple and compound operators help update variable values in C++.
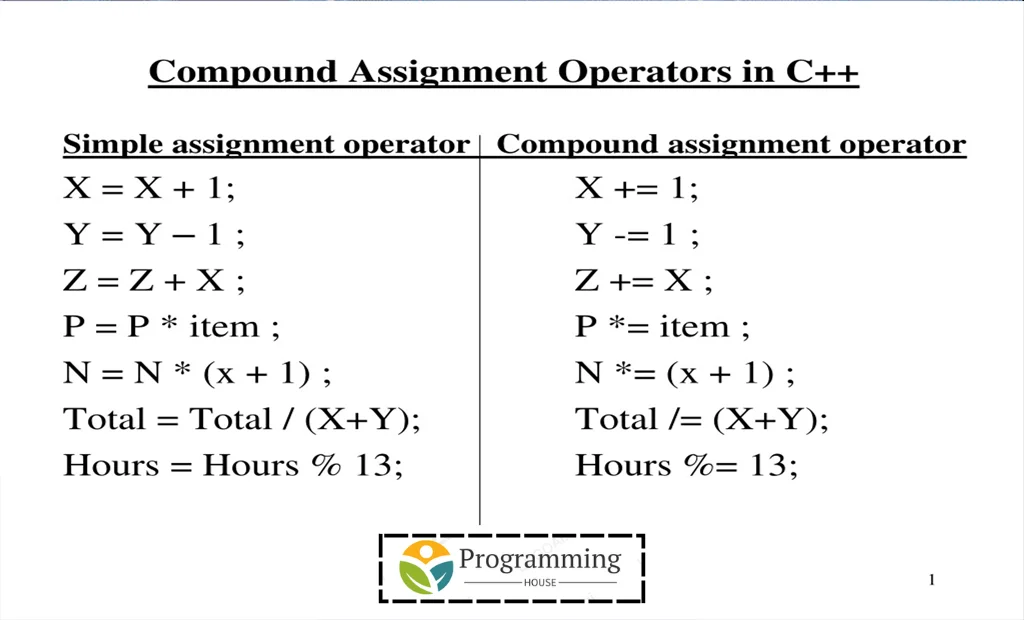
Use Cases for Various Assignment Operators
The assignment operators in C++ have different uses based on the situation. The simple (=) assigns a value to a variable like “x = 5”.
Compound operators like +=, -=, *=, /=, and %= perform math operations and assign the result. For instance, “x += 5” means x = x + 5.
These operators are handy for updating variables with specific calculations. Knowing them is vital for handling variables in C++.
Bitwise Operators and Their Mechanics
Bitwise operations in C++ handle individual bits of operands. These operators work on binary data.
The AND operator (&) compares bits between two operands.
The OR operator (|) combines bits of two operands.
The XOR operator (^) checks the difference between bits of two operands.
The NOT operator (~) flips all bits of an operand.
These operators are useful for modifying or analyzing specific bits of data.
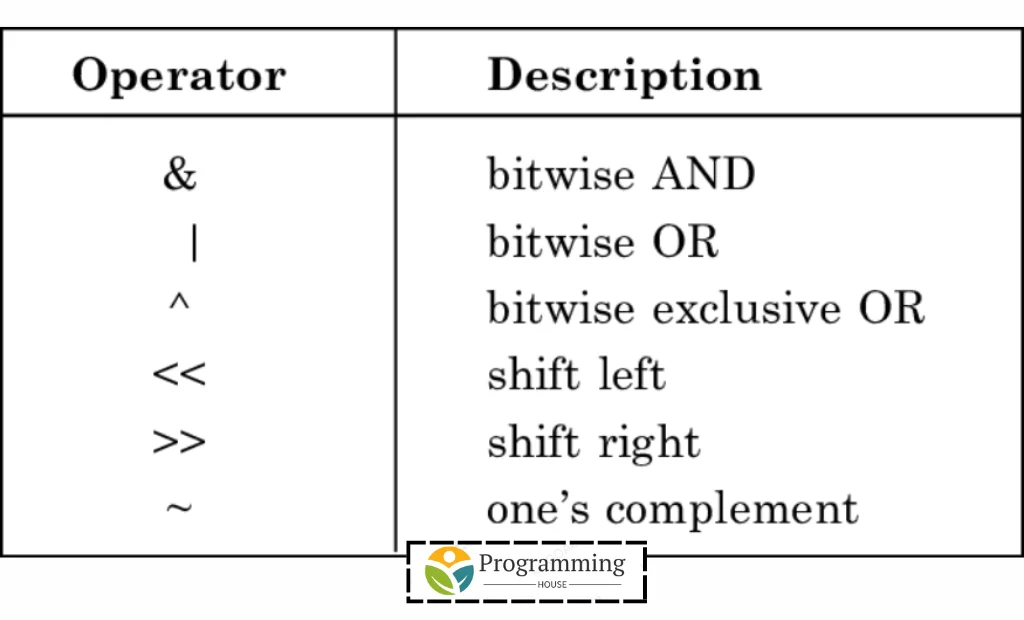
Bitwise AND, OR, XOR, and NOT
The AND operator in C++ compares bits, returning a set bit if both operands have set bits.
The OR operator in C++ combines bits, resulting in a set bit if either operand has a set bit.
The XOR operator in C++ checks for differing bits, setting the result bit accordingly.
The complement operator in C++ flips all bits of the operand to get the ones’ complement.
These operators are frequently utilized to alter single bits in C++.
Shift Operators: Left Shift and Right Shift
The shift operators in C++ move bits left or right.
The left shift operator (<<) shifts bits left by a specified number, discarding leftmost bits and filling rightmost with zeros. For example, “x << 2” shifts x’s bits two positions left.
The right shift operator (>>) shifts bits right by a specified number, discarding rightmost bits and filling leftmost with zeros (unsigned) or sign bit (signed). For instance, “x >> 2” shifts x’s bits two positions right.
The table below summarizes the shift operators:
Operator | Description |
<< | Left shift |
>> | Right shift |
These operators help manage data bits in C++.
Special Operators in C++
C++ also provides special operators that have unique functionality.
The ternary operator (?:) in C is a conditional operator that takes three operands. It evaluates a boolean expression returns the value of the second operand if the expression is true; otherwise, returns the value of the third operand.
The comma operator (,) in C evaluates multiple expressions and returns the value of the last expression.
The sizeof operator in C++ returns the size in bytes of a data type or a variable.
These special operators provide additional flexibility and functionality in C++ programming.
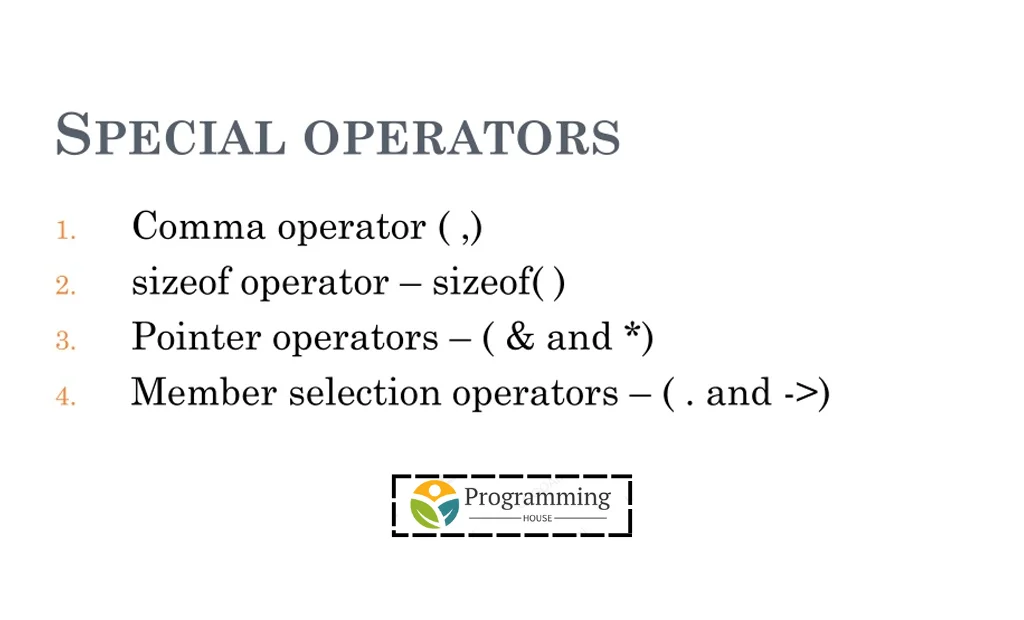
The Ternary Operator Explained
The ternary operator (?:) in C++ is a conditional operator that takes three operands. It evaluates a boolean expression and returns the value of the operand if the expression is true; otherwise, it returns the value of the third operand.
The ternary operator can be used as a shorthand for simple if-else statements. It provides a concise way to make decisions based on a condition and assign values to variables.
Understanding the ternary operator is beneficial for writing compact and readable code in C++.
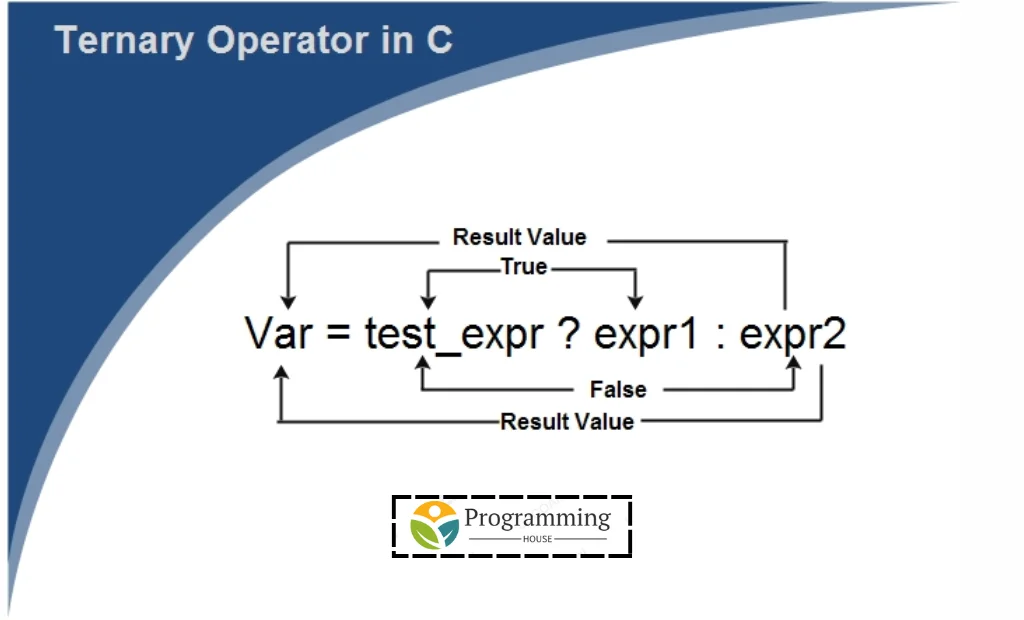
Comma and Size of Operators
The comma operator in C++ evaluates more than one expression and gives the last expression’s value. It helps evaluate expressions one by one, and the result is the last expression’s value.
For instance, in “a = (x++, y++, z++)” example, the comma operator assesses x++, y++, and z++ consecutively. The value of z++ is the final result.
The sizeof operator in C++ shows the size in bytes of a data type or variable. It helps find an object or data type’s size during compilation.
For example, “sizeof(int)” displays the byte size of int, and “sizeof(x)” shows x’s size in bytes.
These operators offer more options and features in C++ programming.
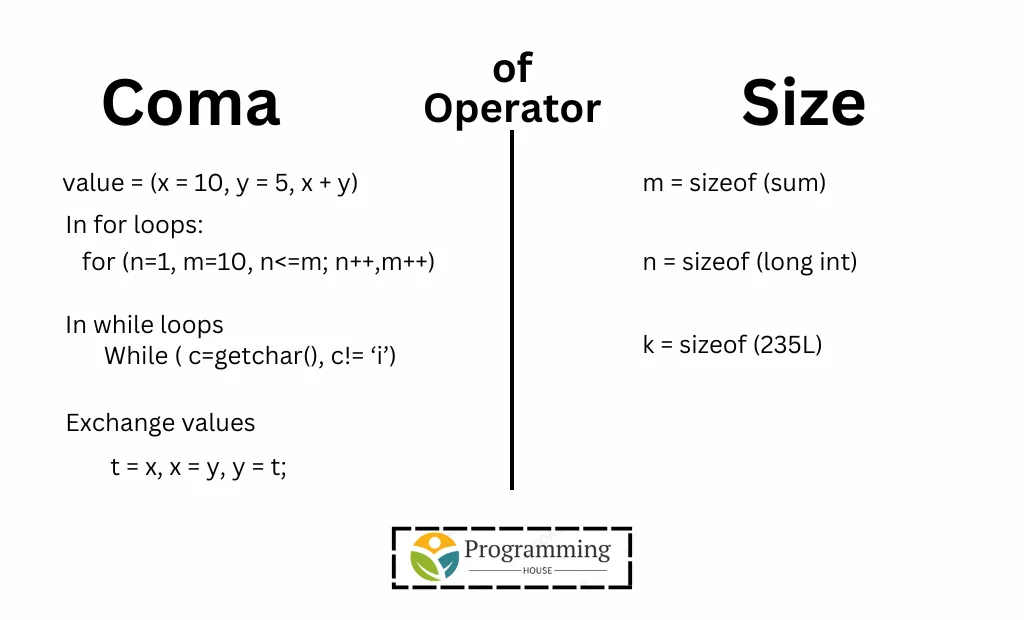
Operator Precedence and Associativity
Operator precedence and associativity in C++ decide the order of operators in an expression. Some have higher precedence. Operators with equal precedence follow their associativity rule.
Precedence decides how terms group and the order of operations. In “a + b * c”, * comes first due to its higher precedence.
Associativity resolves same-precedence operator conflicts. For “+”, left associativity means it’s evaluated left to right in “a + b + c”.
Knowing these rules is vital for coding accurately and predictably in C++.
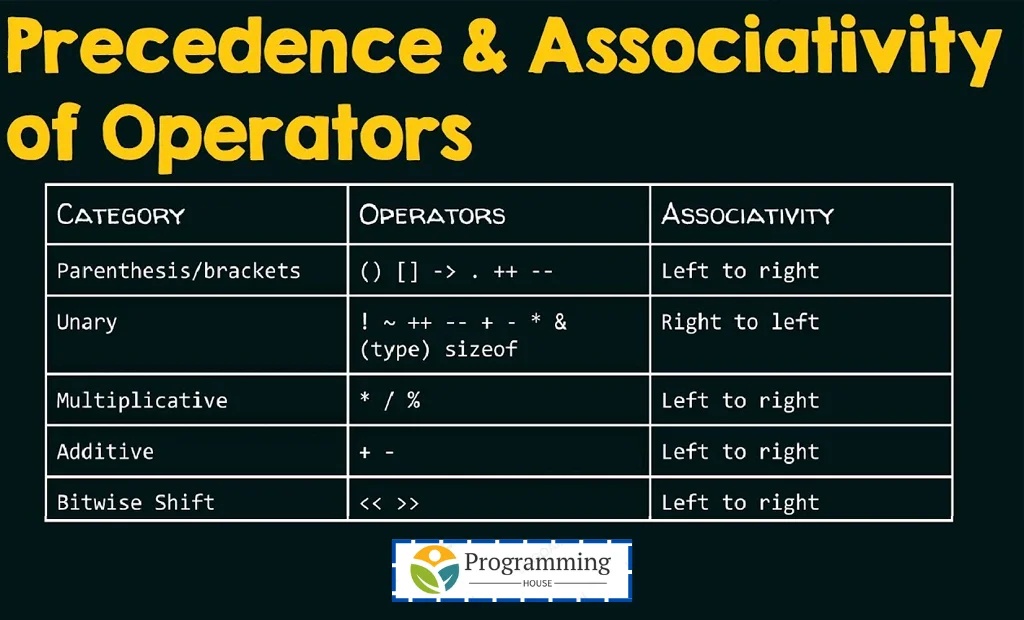
Understanding Operator Precedence
Operator precedence in C++ decides the order of evaluating operators in an expression. It helps group terms and define operations.
In C++, higher precedence operators are evaluated before lower ones. For instance, * is evaluated before + in “a + b * c”.
Parentheses alter the evaluation order by grouping terms; what’s inside parentheses is evaluated first.
Knowing operator precedence in C++ is essential for writing accurate code that produces expected results. It ensures expressions are evaluated correctly and in the intended order.
Associativity of Operators in C++
Associativity in C++ helps with conflicts in expressions with operators of the same precedence. It decides operator evaluation order.
In C++, left associative operators are evaluated left to right, while right associative operators are evaluated right to left.
For instance, the addition operator (+) is left associative. So, in “a + b + c”, it’s “(a + b) + c”.
The assignment operator (=) is right associative. Thus, in “a = b = c”, it’s “a = (b = c)”.
Knowing associativity rules in C++ is crucial for writing precise and consistent code.
Conclusion
Understanding operators in C++ is crucial for mastering the language. Operators like arithmetic and bitwise serve different purposes in programming. Increment, decrement, relational, and assignment operators are essential for loops, variables, decision-making, and data manipulation. The ternary operator provides concise conditional expressions. Knowing operator precedence ensures correct code execution. Exploring operator categories enhances C++ proficiency. Dive into operators to improve coding skills significantly.
Frequently Asked Questions
How do operators differ in C++ compared to other programming languages?
Operators in C++ work like operators in other code languages. They do specific math or logic tasks on data. But, different programming languages may have slightly different rules for certain operators. Thus, it’s vital to grasp the unique guidelines of each language.
Can bitwise operators be used for optimizing code?
Yes, simple operators help optimize code. They work on single bits, making some tasks faster. Yet, use them carefully because they can confuse and lead to mistakes.
What is the significance of operator precedence in complex expressions?
Operator precedence decides the order operators are evaluated. Knowing this is vital for correct expression evaluation. It prevents confusion and guarantees operations occur in the right sequence.
Examples of common mistakes with operators in C++
Using the wrong operator, not assigning correctly, and ignoring precedence in C++ cause errors and issues.