The switch
statement in C++ is a control structure that allows for more efficient branching based on the value of a single variable. It’s particularly useful when you need to compare a variable against multiple constant values. This article will guide you through the basics of the switch
statement with an example program to illustrate its use.
What is a switch
Statement?
The switch statement evaluates the profit of a given expression and executes the corresponding law block guide that value. Unlike the if-else graduated system, that can become awkward when handling multiple environments, the switch charge provides a detergent and more legible form when you have to equate a changing against several likely principles.
Syntax of switch
Statement
Here’s the basic syntax of a switch statement in c++ example program:
switch (expression) {
case constant1:
// code block 1
break;
case constant2:
// code block 2
break;
// you can have any number of case statements
default:
// default code block
}
Key Points to Note:
- Expression: The switch expression is judged before and distinguished accompanying each case fixed.
- Case Constants: Each case is attended by a determined advantage. When the verbalization competitions a case neverending, the code guide that case is performed.
- Break Statement: The break statement is important cause it averts the execution from dropping through to the next case. Without break, all after cases will be performed, although either they competition the verbalization a suggestion of correction.
- Default Case: The default case is possible. It executes when nobody of the case continuous equal the verbalization. Think of it as a replacement alternative.
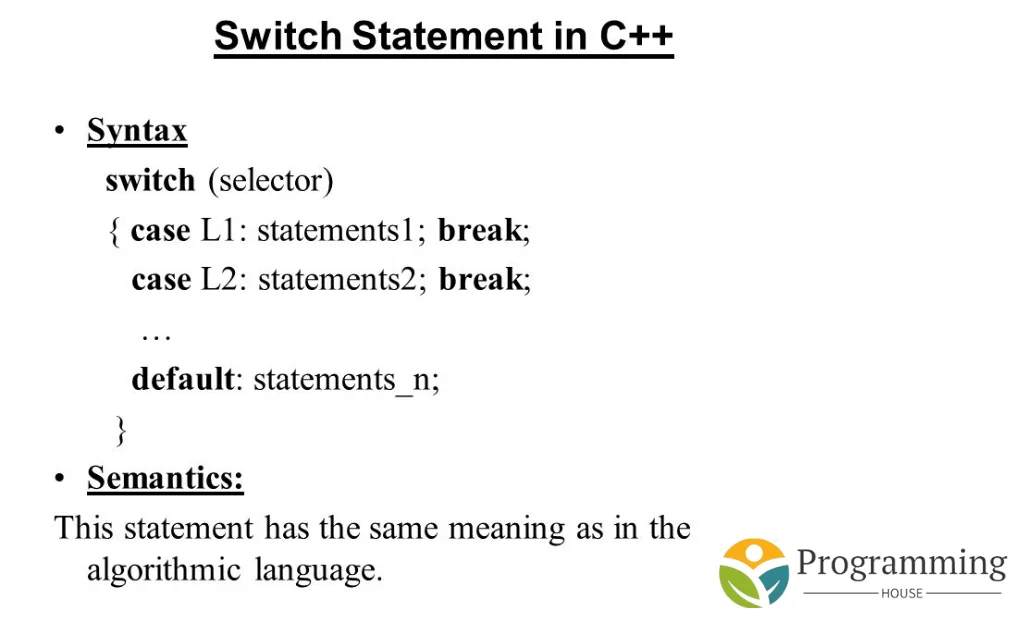
Example Program
Let’s look at a simple switch statement in c++ example program that demonstrates the use of the switch
statement.
#include <iostream>
using namespace std;
int main() {
int day;
cout << "Enter a number (1-7) to represent the day of the week: ";
cin >> day;
switch (day) {
case 1:
cout << "Monday" << endl;
break;
case 2:
cout << "Tuesday" << endl;
break;
case 3:
cout << "Wednesday" << endl;
break;
case 4:
cout << "Thursday" << endl;
break;
case 5:
cout << "Friday" << endl;
break;
case 6:
cout << "Saturday" << endl;
break;
case 7:
cout << "Sunday" << endl;
break;
default:
cout << "Invalid input! Please enter a number between 1 and 7." << endl;
break;
}
return 0;
}
How the Program Works:
- Input: The program prompts the user to enter a number between 1 and 7, which represents a day of the week.
- Switch Statement: The
switch
statement checks the value of the variableday
.- If the value matches
1
, it prints “Monday”. - If the value matches
2
, it prints “Tuesday”, and so on.
- If the value matches
- Default Case: If the user inputs a number outside the range of 1 to 7, the
default
case is executed, printing an error message.
Advantages of Using switch
:
- Readability: The
switch
statement makes the code easier to read and maintain, especially when dealing with multiple conditions. - Efficiency: In many cases,
switch
statements are more efficient than a series ofif-else
statements because the compiler can optimize the jump to the correct case.
Conclusion
The switch statement in c++ example program is a strong tool in C++ compute, particularly when you need to create decisions established a changing’s profit. It is particularly direct when the changing has diversified possible principles, making the law detergent and more manageable than utilizing an thorough if-different ladder. In the model program above, we’ve visualized by means of what to use the switch statement to gain the equivalent day of the period established a consumer input. This fundamental idea maybe extended to many additional uses in C++ place multi-way separate is necessary.