Loops are an important part of C++ control flow because they allow a block of code to be executed numerous times. Among the numerous forms of loops, the posttest loop is most useful in situations when the loop body must execute at least once, regardless of whether the condition is true. This article digs into the complexities of posttest loops in C++, including their syntax, functionality, applications, and best practices. We will also compare them to other loop constructions in C++ to highlight their distinguishing characteristics.
Understanding Loops in C++
Before getting into posttest loops, you should have a fundamental understanding of what loops are and why they are utilized. A loop is a control structure that repeats a block of code as long as a specific condition is met. Loops are required for activities that involve repeated actions, such as iterating over arrays, processing user input, and doing calculations.
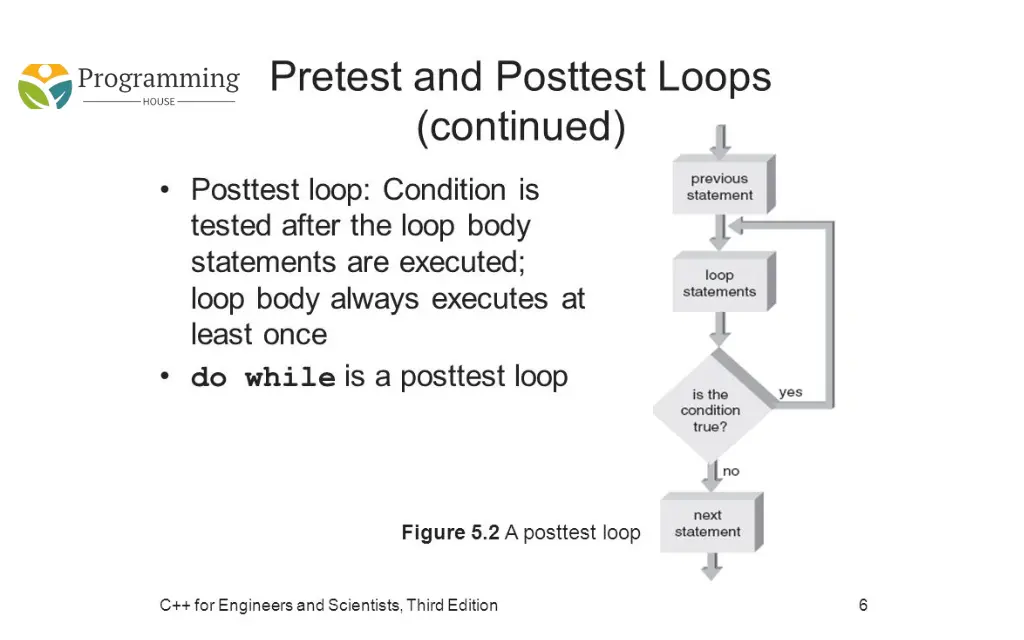
There are three primary types of loops in C++:
- For Loop: A loop that repeats a block of code a known number of times. It’s often used when the number of iterations is predetermined.
- While Loop: A loop that continues to execute as long as a specified condition remains true. It is useful when the number of iterations is not known beforehand.
- Do-While Loop (Posttest Loop): A loop that executes a block of code once before checking the condition. It continues to execute as long as the condition remains true.
The Posttest Loops in C++: Do-While Loop
The Posttest Loops in C++, also known as the do-while loop in C++, is special in that it ensures that the loop body runs at least once. This is in contrast to other loops, which verify the condition before executing the loop body. The do-while loop is very handy when you need to ensure that the code inside the loop executes at least once before any condition is assessed.
Syntax of the Do-While Loop
The syntax of a do-while
loop in C++ is straightforward:
do {
// Statements to be executed
} while (condition);
Here’s a breakdown of the components:
- do: This keyword marks the beginning of the loop.
- Statements to be executed: This is the body of the loop, where the code to be repeated is placed.
- while (condition): After executing the loop body, the condition is checked. If the condition evaluates to true, the loop body executes again. If false, the loop terminates.
Example of a Do-While Loop
Consider a simple example where we want to prompt the user to enter a positive number. If the user enters a negative number, the loop will continue to prompt the user until a positive number is entered.
#include <iostream>
using namespace std;
int main() {
int number;
do {
cout << "Enter a positive number: ";
cin >> number;
} while (number <= 0);
cout << "You entered: " << number << endl;
return 0;
}
In this example, the loop prompts the user to enter a positive number. Even if the user enters a negative number on the first attempt, the loop still runs, ensuring that the prompt is displayed at least once.
Comparing Do-While with Other Loops
To fully appreciate the do-while
loop, it’s helpful to compare it with other loops in C++.
While vs. Do-While Loop
The key difference between a while
loop and a do-while
loop is the timing of the condition check. In a while
loop, the condition is checked before the loop body executes:
while (condition) {
// Statements to be executed
}
In a while
loop, if the condition is false from the start, the loop body may never execute. In contrast, the do-while
loop ensures that the loop body executes at least once:
do {
// Statements to be executed
} while (condition);
Example:
#include <iostream>
using namespace std;
int main() {
int number = -1;
while (number <= 0) {
cout << "Enter a positive number: ";
cin >> number;
}
cout << "You entered: " << number << endl;
return 0;
}
In this while
loop example, if number
is initialized with a positive value, the loop won’t execute at all. However, in a do-while
loop, the body will execute at least once, regardless of the initial value of number
.
Do-While vs. For Loop
A for
loop is generally used when the number of iterations is known beforehand:
for (initialization; condition; increment) {
// Statements to be executed
}
The for loop is more compact and appropriate for scenarios in where you can explicitly define the loop counter’s start, end, and increment. However, unlike the do-while loop, the for loop does not ensure that the loop body is executed at least once, especially if the initial condition is false.
Example:
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 5; i++) {
cout << "Iteration " << i << endl;
}
return 0;
}
This for
loop will iterate five times, but if the initial condition (i <= 5
) was false, the loop would not execute.
Use Cases for Posttest Loops in C++
Posttest loops are particularly useful in scenarios where you need the loop body to execute at least once. Some common use cases include:
- User Input Validation: As seen in the earlier example, posttest loops are ideal for prompting users for input until a valid input is received.
- Menu-Driven Programs: In applications where users need to make selections from a menu, a
do-while
loop can be used to display the menu at least once and keep displaying it until a valid option is selected. - File Processing: When processing files, you might want to read and process data at least once before checking for the end of the file.
- Game Loops: In simple games, the game loop might run at least once to ensure that the game state is updated before checking if the game should continue.
Example: Menu-Driven Program
Here’s an example of a simple menu-driven program that uses a do-while
loop:
#include <iostream>
using namespace std;
int main() {
int choice;
do {
cout << "Menu:" << endl;
cout << "1. Option 1" << endl;
cout << "2. Option 2" << endl;
cout << "3. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
cout << "You selected Option 1" << endl;
break;
case 2:
cout << "You selected Option 2" << endl;
break;
case 3:
cout << "Exiting..." << endl;
break;
default:
cout << "Invalid choice! Please try again." << endl;
}
} while (choice != 3);
return 0;
}
In this example, the menu is displayed at least once, and the program continues to prompt the user until they choose to exit by selecting option 3.
Best Practices for Using Posttest Loops
While the do-while
loop is a powerful tool, it’s important to use it appropriately. Here are some best practices to consider:
- Ensure the Condition Will Eventually Be False: To avoid infinite loops, make sure that the loop condition will eventually evaluate to false. This might involve updating a variable within the loop body.
- Use for Input Validation: Posttest loops are well-suited for input validation scenarios where the loop body must execute at least once.
- Avoid Complex Conditions: To maintain readability, avoid using overly complex conditions in the
while
statement. If the condition is too complicated, consider breaking it down or using helper functions. - Comment Your Code: Since the
do-while
loop is less commonly used than other loops, it’s good practice to include comments explaining why ado-while
loop is necessary in your code. - Be Mindful of Initialization: Ensure that variables used in the loop condition are properly initialized before the loop begins to avoid unexpected behavior.
Common Pitfalls and How to Avoid Them
Even though the do-while
loop is relatively simple, there are some common pitfalls to watch out for:
- Forgetting to Update the Condition: If you forget to update the condition variable inside the loop body, you may end up with an infinite loop. Always ensure that the condition will eventually change to false.
- Using Uninitialized Variables: Since the loop body executes before the condition is checked, using uninitialized variables can lead to undefined behavior.
- Overusing Posttest Loops: While
do-while
loops are useful, they are not always the best choice. Overusing them can lead to less readable code. Use them only when the situation clearly calls for a loop that must execute at least once.
Advanced Topics of Posttest Loops in C++
Nested Do-While Loops
Like other loops, do-while
loops can be nested. This means you can place one do-while
loop inside another. Nested