Understanding Nested If-Else Statements in Programming
In programming, decision-making structures allow developers to control the flow of execution based on certain conditions. One of the most commonly used decision-making structures is the if-else
statement. When you need to evaluate multiple conditions, you can use a structure known as nested if-else statements.
What is a Nested If-Else Statement?
A nested if-else statement is an if-else
statement within another if-else
statement. This allows for more complex decision-making processes where one set of conditions can lead to another set of conditions that must be evaluated.
Basic Structure:
if condition1:
# Executes when condition1 is true
if condition2:
# Executes when condition2 is true and condition1 is true
else:
# Executes when condition2 is false and condition1 is true
else:
# Executes when condition1 is false
if condition3:
# Executes when condition3 is true and condition1 is false
else:
# Executes when condition3 is false and condition1 is false
How Does It Work?
- The program first checks
condition1
. Ifcondition1
is true, the program moves inside the first block of code. - Inside this block,
condition2
is evaluated. Ifcondition2
is true, a specific block of code executes. If false, theelse
block associated withcondition2
is executed. - If
condition1
is false, the program skips to theelse
part, wherecondition3
is evaluated, leading to similar branching.
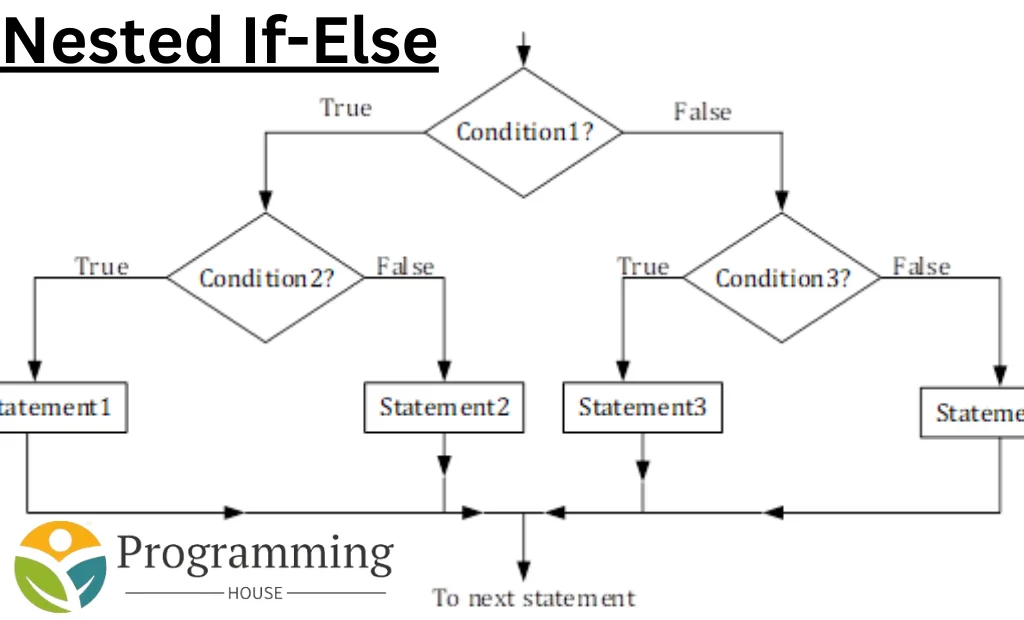
Example of Nested If-Else in Python
Consider a scenario where you want to categorize a person based on their age:
age = 25
if age < 13:
print("Child")
else:
if age < 20:
print("Teenager")
else:
if age < 60:
print("Adult")
else:
print("Senior")
Explanation:
- The program first checks if
age
is less than 13. If true, it prints “Child.” - If
age
is not less than 13, it moves to the next condition, checking ifage
is less than 20. If true, it prints “Teenager.” - If neither of the first two conditions is true, the program checks if
age
is less than 60. If true, it prints “Adult.” - Finally, if all previous conditions are false, it prints “Senior.”
Simplifying with elif
While nested if-else statements are powerful, they can sometimes become cumbersome and difficult to read. Most programming languages offer an alternative called elif
(short for “else if”) to simplify this structure.
Here’s the same example rewritten using elif
:
pythonCopy codeage = 25
if age < 13:
print("Child")
elif age < 20:
print("Teenager")
elif age < 60:
print("Adult")
else:
print("Senior")
This version is more readable and achieves the same outcome with fewer lines of code.
Practical Applications
Nested if-else statements are particularly useful when:
- You have multiple layers of conditions that depend on each other.
- The outcome of one condition changes the subsequent conditions that need to be checked.
- Complex decision-making logic is required.
For instance, in game development, nested if-else statements can determine the outcome of a player’s actions based on multiple in-game conditions.
Best Practices
- Readability: Nesting can quickly make code hard to read. Use
elif
where possible or refactor the code to reduce the depth of nesting. - Maintainability: Keep nested if-else statements simple. If you find yourself nesting more than two or three levels deep, consider breaking down the logic into functions.
- Performance: Although modern compilers and interpreters are efficient, deeply nested if-else statements can impact performance, especially in loops. Always be mindful of optimizing where necessary.
Conclusion
Nested if-else statements are a strong tool in a someone proficient at computers’s armory for management complex administrative processes. While they allow for elaborate rationale and dependent flows, it’s main to use ruling class judiciously to claim law readability and effectiveness. By understanding when and by what method to use nested if-else statements, you can print more direct and maintainable law.