Introduction
Programming’s core idea of loops enables programmers to continually run a block of code in response to a predefined condition. The while loop and the do-while loop are two popular loop constructions that frequently cause confusion among novices among the different types of loops available. Despite their commonalities, they differ in important ways that affect how and when each should be utilized. This article explores the syntax, features, applications, and main distinctions between the while and do-while loops in great detail. You will have a thorough comprehension of these loops and know how to use them successfully in your programming projects by the end of this study.
What is a Loop?
Before delving into the intricacies of the while and do-while loops, it is imperative to comprehend the fundamental idea of loops in programming. When a given condition is met, a loop is a control structure that continually runs a block of code. Loops are effective tools for processing data, automating repetitive operations, and managing a program’s flow.
There are several types of loops in programming including:
- For Loop: Typically used when the number of iterations is known.
- While Loop: Executes as long as a condition is true, with the condition checked before each iteration.
- Do-While Loop: Similar to the while loop, but with the condition checked after the code block has executed.
Each loop type has its advantages and is suited to different scenarios, but in this article, we will focus on the while
and do-while
loops.
The While
Loop
Syntax and Structure
The while
loop is one of the simplest and most commonly used loops in programming. Its basic syntax is as follows:
while (condition) {
// Code to execute
}
Here’s a breakdown of the syntax:
- Condition: This is a Boolean expression that evaluates. The loop will continue to execute as long as this condition remains true.
- Code Block: The code within the curly braces
{}
is the body of the loop.
How It Works
The condition is first assessed in the while loop. The body of the loop runs if the condition is true. The state is assessed once again following the execution of the corpse. The loop repeats itself if the condition remains true. Until the condition is no longer true, this process is repeated.
Example 1: Basic While
Loop
counter = 0
while counter < 5:
print("Counter:", counter)
counter += 1
In this example:
- The loop initializes with
counter
set to 0. - The condition
counter < 5
is checked. Since it’s true, the loop prints the current value ofcounter
. - The
counter
is then incremented by 1. - The loop continues to run until
counter
reaches 5, at which point the conditioncounter < 5
becomes false, and the loop terminates.
Example 2: Infinite While
Loop
while True:
print("This will run forever")
In this example, the condition is always True
, creating an infinite loop. Such loops are generally avoided unless you have a specific reason to keep a process running indefinitely, typically with a break condition inside the loop.
Use Cases for While
Loop
- User Input Validation: Continuously prompt a user for input until they provide a valid response.
- Polling Systems: Check for the status of an external system or process until a specific condition is met.
- Game Loops: Keep a game running until a player wins, loses, or decides to quit.
The while
loop is useful when the number of iterations is not known beforehand and depends on dynamic conditions that change during runtime.
The Do-While
Loop
Syntax and Structure
The do-while
loop is similar to the while
-loop but with a key difference in how the condition is evaluated. Its basic syntax is as follows:
do {
// Code to execute
} while (condition);
Here’s a breakdown of the syntax:
- Code Block: The code within the curly braces
{}
is the body of the loop. - Condition: This Boolean expression is checked after the code block has executed. If the condition is true, the loop continues to execute. If false, the loop terminates.
How It Works
The do-while
loop executes the code block once before checking the condition. After the initial execution, it evaluates the condition. If the condition is true, the loop repeats; if false, it stops. This guarantees that the loop’s body is executed at least once, regardless of the condition.
Example 1: Basic Do-While
Loop
counter = 0
do {
print("Counter:", counter)
counter += 1
} while (counter < 5);
In this example:
- The loop starts by printing the
counter
, which is initially 0. - The
counter
is then incremented by 1. - The condition
counter < 5
is checked after the first iteration. - The loop continues until the
counter
reaches 5, similar to thewhile
loop, but with the key difference that the loop body executes before the condition is evaluated.
Example 2: User Prompt Example
do {
user_input = input("Enter 'yes' to continue: ")
} while (user_input != "yes");
In this example:
- The loop asks the user to input “yes” to continue.
- The loop’s body runs at least once, ensuring the user is prompted at least one time.
- If the user does not enter “yes”, the loop repeats.
Use Cases for Do-While
Loop
- Menu-Driven Programs: Display a menu to the user and continue to prompt until a valid option is selected.
- Data Processing: Perform an action on data and then check if further processing is needed.
- Retry Mechanisms: Attempt an action and retry if it fails, continuing until success or a maximum number of attempts is reached.
The do-while
loop is particularly useful when you need the code to run at least once, regardless of whether the condition is initially true or false.
Key Differences B/w While
and Do-While
Loops
While both loops are designed for repetitive execution of code blocks, their differences can significantly impact how and when they are used. Below are the key differences:
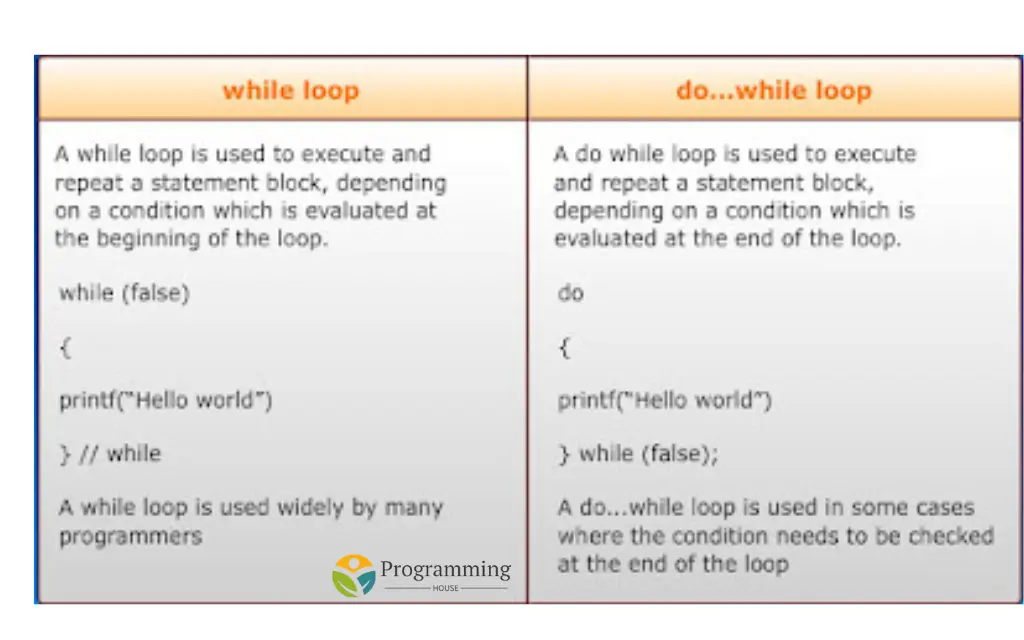
- Condition Evaluation Timing:
- While Loop: The condition is evaluated before the loop’s body executes. If the condition is false initially, the loop’s body never executes.
- Do-While Loop: The condition is evaluated after the loop’s body executes, ensuring the loop’s body runs at least once.
- Guaranteed Execution:
- While Loop: No guaranteed execution. If the condition is false at the start, the loop’s body will not run at all.
- Do-While Loop: Guarantees at least one execution of the loop’s body, regardless of the initial condition.
- Syntax and Readability:
- While Loop: Simpler and more straightforward syntax, making it easier to read and understand in scenarios where a pre-condition check is necessary.
- Do-While Loop: Slightly more complex due to the condition being placed after the loop’s body, which might make it less intuitive for beginners.
- Common Use Cases:
- While Loop: Best used when the number of iterations depends on a condition that might not be met initially.
- Do-While Loop: Ideal when at least one execution of the loop is required, such as when presenting a menu or prompt that must appear at least once.
Practical Examples and Comparison
Let’s compare while
and do-while
loops through practical scenarios:
Scenario 1: Password Validation
Imagine a scenario where a user must enter a password correctly to proceed.
- While Loop Implementation:
password = ""
while password != "secret":
password = input("Enter your password: ")
print("Access granted.")
- Do-While Loop Implementation:
do {
password = input("Enter your password: ")
} while (password != "secret");
print("Access granted.")
In this case:
- The
do-while
loop is preferable as it guarantees the user is prompted at least once, which is expected behavior in this scenario.
Scenario 2: Sensor Data Monitoring
Consider a scenario where you monitor sensor data and take action only if certain conditions are met.
- While Loop Implementation:
sensor_value = get_sensor_value()
while sensor_value < threshold:
sensor_value = get_sensor_value()
// Process sensor data
- Do-While Loop Implementation:
do {
sensor_value = get_sensor_value()
// Process sensor data
} while (sensor_value < threshold);
In this case:
- The
while
loop is more appropriate if the initial sensor value needs to be checked before processing. - The
do-while
loop might be used if processing must occur at least once before making a decision.
Performance Considerations
From a performance perspective, both while
and do-while
loops are generally equivalent in terms of efficiency. However, the context of their usage can impact overall program logic and flow. It’s essential to choose the
loop that best matches the logical requirements of your program, ensuring that your code is not only efficient but also clear and maintainable.
Conclusion
Writing effective and fast code requires an understanding of the distinctions between the while and do-while loops. Although the goal of both loops is to repeat code based on a condition, they behave differently in your programs depending on when the condition is tested.
When you need to verify a condition before carrying out the body of the loop, the while loop works perfectly.
When the body of the loop needs to run at least once, regardless of the condition, the do-while loop works well.
It’s important to comprehend the particular needs of your program and the desired behavior before selecting one of these loops. You can build more adaptable, dependable, and comprehensible code and become a more proficient programmer by grasping these ideas.