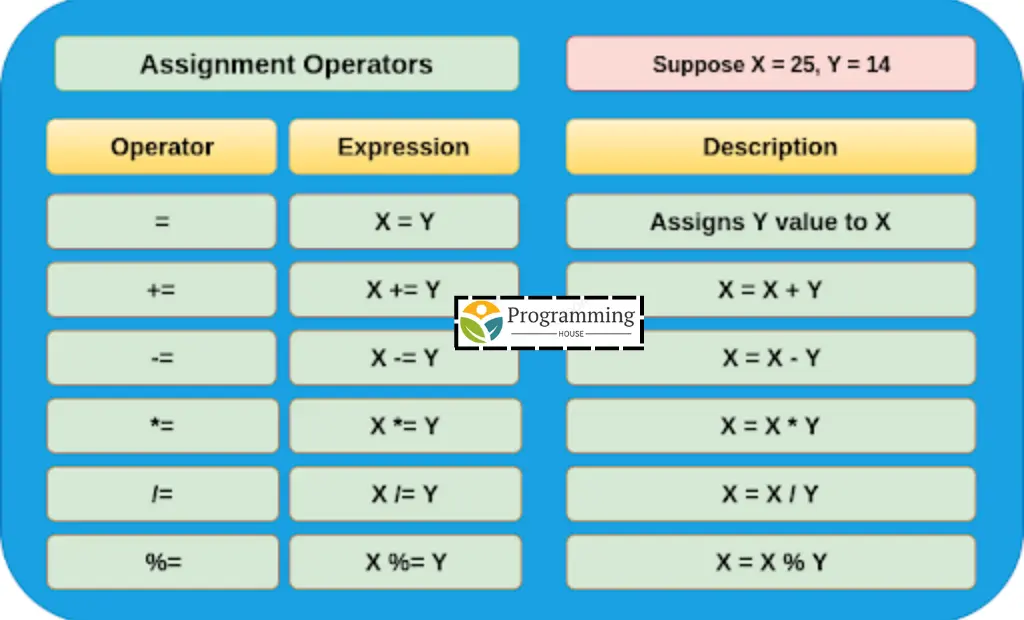
Key Highlights
- Assignment operators are used to assign values to variables in C programming.
- The simple assignment operator “=” assigns a value on the side to the variable on the left side.
- Compound operators like “+=” and “-=” perform operation and then assign the result the variable.
- Assignment operators have lower precedence than other operators in C.
- Understanding assignment operators is crucial for manipulating variables and performing calculations in C.
Introduction
In C programming, assignment operators assign values to variables. Programmers use “=” for this. The left side is a variable, the right side a value or expression.
The “=” operator in C assigns the right value to the left variable. For instance, “x = 5;” sets x as 5.
C also has compound assignment operators like “+=” that combine an operation and assignment. These operators calculate using both sides and assign the result to the left variable.
Knowing assignment operators is crucial for variable manipulation in C programming. Different operators help streamline code and assign values effectively.
Exploring C Programming Assignment Operators
Assignment operators in C are used to give values to variables. These operators modify variable values in expressions. C has various assignment operators, such as “=”, “+=”, “-=”, “*=”, “/=”, “%=”, “<<=”, “>>=”, “&=”, “|=”, and “^=”. They help efficiently assign and change values. These operators work with various data types and can do arithmetic and bitwise operations during assignment.
1. Simple Assignment Operator (=)
The basic “=” operator in C programming assigns the value on the right to the variable on the left. In an example like int x = 5;
, 5 goes to variable x using “=” operator. The variable x then holds the value 5. This operator suits integers, floats, characters, and custom data types but requires matching data types. If not matched, an error occurs. It’s often used to set or change values in programs.
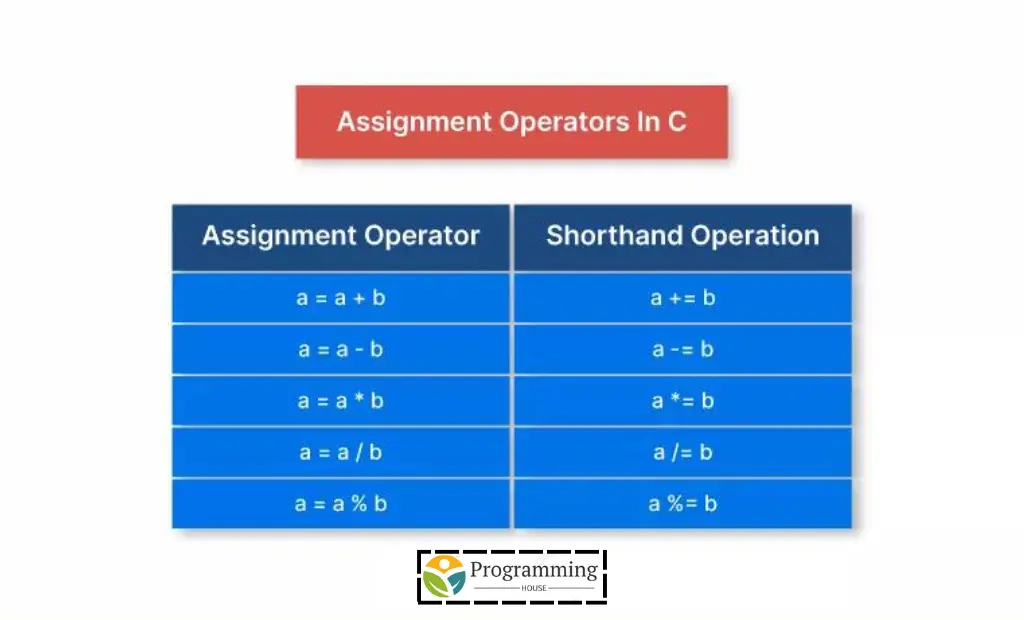
2. Addition Assignment Operator (+=)
The addition assignment operator “+=” is a compound assignment operator in C programming. It combines the addition operation with the assignment operation. It adds the value on the right side to the current value of the variable on the left side and assigns the result back to the variable on the left side.
For example:
int x = 5; x += 3;
In the above example, the current value of the variable x is 5. The addition assignment operator adds the value 3 to the current value of x, resulting in x being updated to 8.
The addition assignment operator can be used with different data types, such as integers, floats, and characters. It provides a concise way to perform addition and assignment in a single statement. It is particularly useful when updating variables in loops or incrementing/decrementing variables.
3. Subtraction Assignment Operator (-=)
The subtraction assignment operator “-=” is a compound assignment operator in C programming. It combines the subtraction operation with the assignment operation. It subtracts the value on the right side from the current value of the variable on the left side and assigns the result back to the variable on the left side.
For example:
int x = 10; x -= 3;
In the above example, the current value of the variable x is 10. The subtraction assignment operator subtracts the value 3 from the current value of x, resulting in x being updated to 7.
The subtraction assignment operator is commonly used to decrement variables by a specific value. It provides a concise way to perform subtraction and assignment in a single statement.
4. Multiplication Assignment Operator (*=)
The multiplication assignment operator “*=” is a compound assignment operator in C programming. It combines the multiplication operation with the assignment operation. It multiplies the value on the right side with the current value of the variable on the left side and assigns the result back to the variable on the left side.
For example:
int x = 5; x *= 2;
In the above example, the current value of the variable x is 5. The multiplication assignment operator multiplies the value 2 with the current value of x, resulting in x being updated to 10.
The multiplication assignment operator is commonly used to multiply variables by a specific value. It provides a concise way to perform multiplication and assignment in a single statement.
5. Division Assignment Operator (/=)
The division assignment operator “/=” is a compound assignment operator in C programming. It combines the division operation with the assignment operation. It divides the current value of the variable on the left side by the value on the right side and assigns the quotient back to the variable on the left side.
For example:
int x = 10; x /= 3;
In the above example, the current value of the variable x is 10. The division assignment operator divides the current value of x by the value 3, resulting in x being updated to 3.
The division assignment operator performs integer division, which means it discards the remainder. If you want to obtain the remainder, you can use the modulus assignment operator (%=).
The division assignment operator is commonly used to divide variables by a specific value. It provides a concise way to perform division and assignment in a single statement.
6. Modulus Assignment Operator (%=)
The modulus assignment operator “%=” is a compound assignment operator in C programming. It combines the modulus operation with the assignment operation.
For example:
int x = 10; x %= 3;
In the above example, the current value of the variable x is 10. The modulus assignment operator divides the current value of x by the value 3 and assigns the remainder (1) back to x.
The modulus assignment operator is commonly used to obtain the remainder of division. It provides a concise way to perform modulus operation and assignment in a single statement.
7. Left Shift Assignment Operator (<<=)
The left shift assignment operator “<<=” is a compound assignment operator in C . It combines the left shift operation with the assignment operation. It shifts the bits of the variable on the left side to the left by the number of positions specified by the value on the right side and assigns the result back to the variable on the left side.
For example:
int x = 10; x <<= 2;
In the above example, the current value of the variable x is 10. The left shift assignment operator shifts the bits of x to the left by 2 positions, resulting in x being updated to 40.
The left shift assignment operator is commonly used in bitwise operations to manipulate individual bits of a variable. It provides a concise way to perform left shift operation and assignment in a single statement.
8. Right Shift Assignment Operator (>>=)
The right shift assignment operator “>>=” is a compound assignment operator in C programming. It combines the right shift operation with the assignment operation. It shifts the bits of the variable on the left side to the right by the number of positions specified by the value on the right side and assigns the result back to the variable on the left side.
For example:
int x = 10; x >>= 2;
In the above example, the current value of the variable x is 10. The right shift assignment operator shifts the bits of x to the right by 2 positions, resulting in x being updated to 2.
The right shift assignment operator is commonly used in bitwise operations to manipulate individual bits of a variable. It provides a concise way to perform right shift operation and assignment in a single statement.
9. Bitwise AND Assignment Operator (&=)
The bitwise AND assignment operator “&=” is a compound assignment operator . It combines the bitwise AND operation with the assignment operation. It performs a bitwise AND operation between the variable on the left side and the value on the right side and assigns the result back to the variable on the left side.
For example:
int x = 5; x &= 3;
In the above example, the current value of the variable x is 5. The bitwise AND assignment operator performs a bitwise AND operation between x and 3, resulting in x being updated to 1.
The bitwise AND assignment operator is commonly used in bitwise operations to perform logical AND operations on individual bits of a variable. It provides a concise way to perform bitwise AND operation and assignment in a single statement.
10. Bitwise OR Assignment Operator (|=)
The bitwise OR assignment operator “|=” is a compound assignment operator in C programming. It combines the bitwise OR operation with the assignment operation. It performs a bitwise OR operation between the variable on the left side and the value on the right side and assigns the result back to the variable on the left side.
For example:
int x = 5; x |= 3;
In the above example, the current value of the variable x is 5. The bitwise OR assignment operator performs a bitwise OR operation between x and 3, resulting in x being updated to 7.
The bitwise OR assignment operator is commonly used in bitwise operations to perform logical OR operations on individual bits of a variable. It provides a concise way to perform bitwise OR operation and assignment in a single statement.
11. Bitwise XOR Assignment Operator (^=)
The bitwise XOR assignment operator “^=” is a compound assignment operator in C programming. It combines the bitwise XOR operation with the assignment operation. It performs a bitwise XOR operation between the variable on the left side and the value on the right side and assigns the result back to the variable on the left side.
For example:
int x = 5; x ^= 3;
In the above example, the current value of the variable x is 5. The bitwise XOR assignment operator performs a bitwise XOR operation between x and 3, resulting in x being updated to 6.
The bitwise XOR assignment operator is commonly used in bitwise operations to perform logical XOR operations on individual bits of a variable. It provides a concise way to perform bitwise XOR operation and assignment in a single statement.
Understanding the Use of Assignment Operators in C
Assignment operators are an important part of the C programming language. They allow programmers to assign values to variables and modify variable values efficiently. By using assignment operators, programmers can write concise and readable code. Assignment operators also play a role in improving code efficiency, as they allow for the execution of multiple operations in a single statement. Understanding the use of assignment operators in C is crucial for writing efficient and readable code.
How Assignment Operators Enhance Code Readability
Assignment operators enhance code readability in C programming. By using assignment operators, programmers can write concise and self-explanatory code. Instead of writing multiple lines of code to assign values to variables, assignment operators allow for the execution of assignment and operation in a single statement. This reduces the number of lines of code and makes the code more readable. Assignment operators also make the code more maintainable, as the intent of the code is clear and the logic is easier to understand. Overall, assignment operators improve code readability and make the code more efficient and concise.
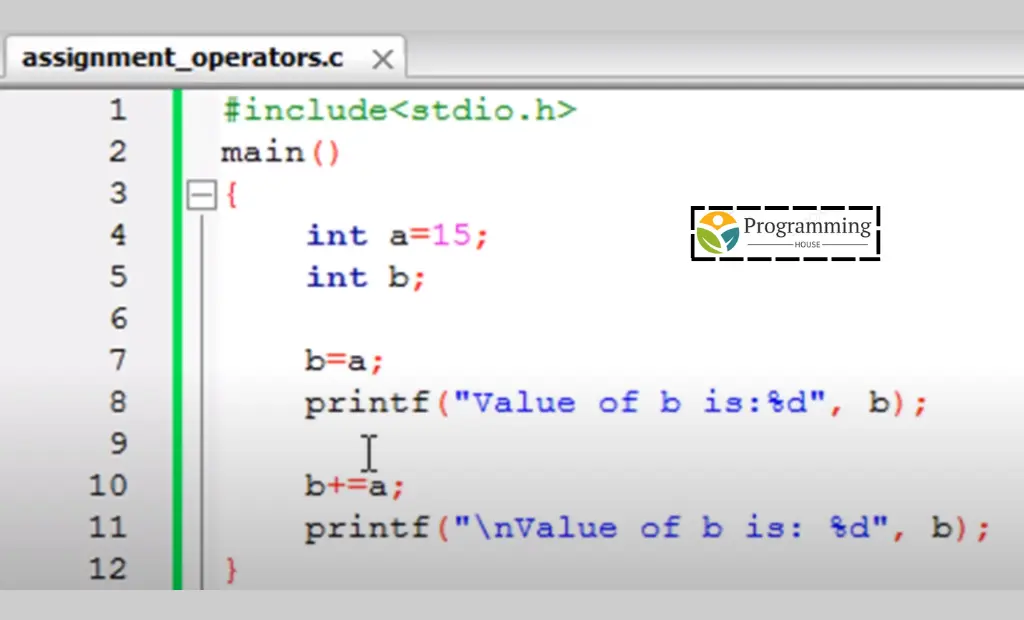
The Efficiency of Using Compound Assignment Operators
Using compound assignment operators in C programming can improve code efficiency. Compound assignment operators combine an operation with the assignment operation, allowing for the execution of multiple operations in a single statement. This reduces the number of lines of code and improves code efficiency. By using compound assignment operators, programmers can write more concise code and reduce the execution time of the program. Additionally, compound assignment operators make the code more readable and maintainable, as the intent of the code is clear and the logic is easier to understand. Overall, using compound assignment operators enhances code efficiency and improves the overall performance of the program.
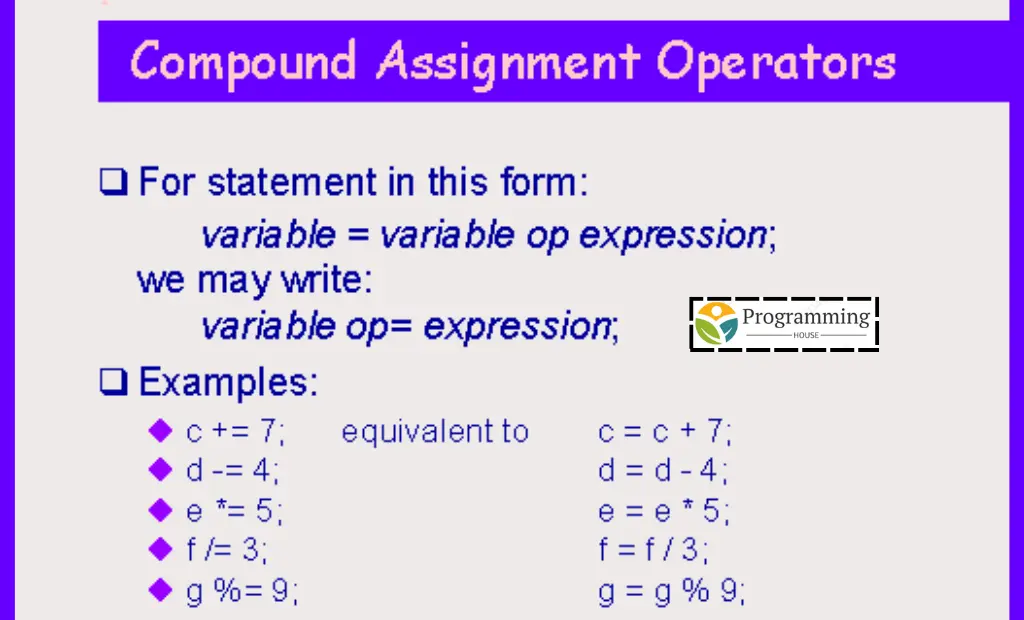
Practical Examples of Assignment Operators in C Programming
Practical examples of assignment operators in C programming can help illustrate their usage and benefits. These examples demonstrate how assignment operators can be used to assign values to variables, perform arithmetic operations, and update variable values. By applying assignment operators in real-world scenarios, programmers can understand their practical applications and improve their understanding of C programming concepts. Practical examples also provide hands-on experience and reinforce the knowledge gained from studying the theory of assignment operators. Let’s explore some practical examples to illustrate the use of assignment operators in C programming.
Example Demonstrating Simple Assignment Operator
Here is an example that demonstrates the use of the simple assignment operator in C programming:
#include <stdio.h> int main() { int x; x = 5; printf("The value of x is %d\n", x); return 0;
In this example, we declare a variable x of the type int. We then assign the value 5 to x using the simple assignment operator “=”.
The printf() function is used to display the value of x on the console. The output of this program will be “The value of x is 5”.
This example showcases how the simple assignment operator can be used to assign a value to a variable in C programming. It demonstrates the basic usage and functionality of the assignment operator.
Implementing Compound Assignment Operators in Loops
Compound assignment operators can be particularly useful when used in loops in C programming. They can simplify and optimize code by combining assignment and operation in a single statement. Here is an example demonstrating the implementation of compound assignment operators in loops:
#include <stdio.h>
int main() {
int i;
int sum = 0;
for (i = 1; i <= 10; i++) {
sum += i;
}
printf ("The sum of no from 1 to 10 is %d\n", sum);
return 0
In this example, we use the compound assignment operator “+=” to calculate the sum of numbers from 1 to 10. The loop iterates from 1 to 10, and in each iteration, the value of i is added to the variable sum using the “+=” operator.
The printf() function is used to display the sum of the numbers from 1 to 10 on the console. The output of this program will be “The sum of numbers from 1 to 10 is 55”.
This example demonstrates how compound assignment operators can simplify code and perform calculations efficiently in loops.
Conclusion
In essence, mastering C programming assignment operators is fundamental for code efficiency and readability. Understanding the nuances of simple (=) to complex bitwise XOR (^=) operators is key to optimizing your code. By implementing compound assignment operators effectively, you streamline your programming process and enhance code comprehension. Explore practical examples and frequently asked questions to solidify your grasp on these operators. Embrace the power of assignment operators in C programming to elevate your coding skills to new heights effortlessly.
Frequently Asked Questions
What is the difference between = and == in C?
The “=” operator is the simple assignment operator in C, used to assign a value to a variable. On the other hand, the “==” operator is the equality operator in C, used to compare two values for equality. While “=” assigns a value to a variable, “==” tests whether two values are equal. For example, “x = 5;” assigns the value 5 to the variable x, while “if (x == 5)” tests whether the value of x is equal to 5.