Types of Inheritance in C++ is individual of the cornerstones of Object-Oriented Programming (OOP), acknowledging rule reusability and logical hierarchic connections middle from two points classes. In C++, inheritance allows individual class (known as the derivative or baby class) to receive characteristics and conducts (like data appendages and part of a group functions) from another class (famous as the base or person class). This method form C++ a powerful speech for establishing standard, reasonable, and extensile programs. Let’s explore the various types of heritage that C++ offers and believe by what method each everything.
1. Types of Inheritance in C++ Single Inheritance
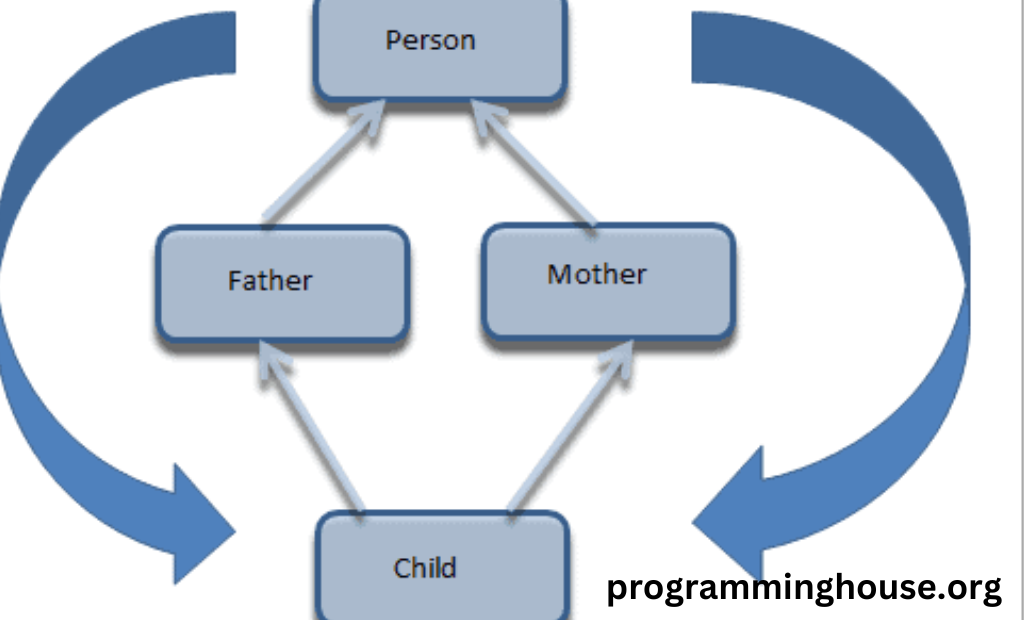
Single inheritance is the most natural form of inheritance in C++. In this type, a class derives or inherits from alone base class. This wealth that the copied class has approach to the features and plans of individual person class only. It’s like a person-youth connection place individual minor inherits from a alone person.
Example:
class Animal {
public:
void eat() {
std::cout << "Eating..." << std::endl;
}
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Barking..." << std::endl;
}
};
int main() {
Dog dog;
dog.eat(); // Inherited from Animal
dog.bark(); // Defined in Dog
return 0;
}
Here, the class Dog
inherits from Animal
. The dog can both bark (its own behavior) and eat (inherited behavior).
2. Multiple Inheritance Types of Inheritance in C++
In multiple inheritance, a class that can b derived form more before individual class. This can frequently cause complexities, to a degree the “jewel question” place a derived class can take over from diversified classes that themselves take over from a common base class. However, when secondhand correctly, diversified bequest allows a class to connect functionalities from differing beginnings.
Example:
class Vehicle {
public:
void move() {
std::cout << "Moving..." << std::endl;
}
};
class Engine {
public:
void start() {
std::cout << "Starting engine..." << std::endl;
}
};
class Car : public Vehicle, public Engine {
public:
void honk() {
std::cout << "Honking..." << std::endl;
}
};
int main() {
Car car;
car.move(); // From Vehicle
car.start(); // From Engine
car.honk(); // From Car
return 0;
}
In this case, the Car
class inherits both the ability to move (from Vehicle
) and to start the engine (from Engine
), creating a fully functional object that combines behaviors.
3. Multilevel Inheritance Types of Inheritance in C++
Multilevel inheritance occurs when a class derives from another derived class. It creates a chain where one class passes down its properties to another, which in turn passes them to a further derived class. This is akin to a grandparent-parent-child relationship.
Example:
class LivingBeing {
public:
void breathe() {
std::cout << "Breathing..." << std::endl;
}
};
class Animal : public LivingBeing {
public:
void eat() {
std::cout << "Eating..." << std::endl;
}
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Barking..." << std::endl;
}
};
int main() {
Dog dog;
dog.breathe(); // Inherited from LivingBeing
dog.eat(); // Inherited from Animal
dog.bark(); // Defined in Dog
return 0;
}
In this example, the class Dog
inherits from Animal
, which in turn inherits from LivingBeing
. Thus, the dog can breathe, eat, and bark.
4. Hierarchical Inheritance
In hierarchical inheritance, multiple derive classes inherit from the same parent class. This is like a parent having several children, all of whom inherit common traits, but each might also have its own specific features.
Example:
class Animal {
public:
void eat() {
std::cout << "Eating..." << std::endl;
}
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Barking..." << std::endl;
}
};
class Cat : public Animal {
public:
void meow() {
std::cout << "Meowing..." << std::endl;
}
};
int main() {
Dog dog;
Cat cat;
dog.eat(); // Inherited from Animal
dog.bark(); // Defined in Dog
cat.eat(); // Inherited from Animal
cat.meow(); // Defined in Cat
return 0;
}
Here, both Dog
and Cat
inherit from the base class Animal
, gaining the ability to eat, but each also has its own distinct behavior.
5. Hybrid Inheritance
Hybrid inheritance is a mixture of more than one type of inheritance. This may involve multiple inheritance and multilevel inheritance working together. Hybrid inheritance can introduce complexity, but it provides flexibility for designing more intricate systems.
Example:
class Engine {
public:
void start() {
std::cout << "Engine starting..." << std::endl;
}
};
class Vehicle {
public:
void move() {
std::cout << "Vehicle moving..." << std::endl;
}
};
class Car : public Vehicle, public Engine {
public:
void honk() {
std::cout << "Honking..." << std::endl;
}
};
class SportsCar : public Car {
public:
void boost() {
std::cout << "Boosting speed..." << std::endl;
}
};
int main() {
SportsCar sportscar;
sportscar.start(); // From Engine
sportscar.move(); // From Vehicle
sportscar.honk(); // From Car
sportscar.boost(); // Defined in SportsCar
return 0;
}
In this hybrid case, SportsCar
inherits from Car
(which itself inherits from both Vehicle
and Engine
), allowing it to combine the abilities of multiple parent classes.
Conclusion
Types of Inheritance in C++ in C++ is a powerful tool that promotes code reusability and a cleaner, more organized code structure. Depending on the scenario, you can use single, multiple, multilevel, hierarchical, or hybrid inheritance to build flexible and scalable applications. However, with this power comes the responsibility to design systems carefully to avoid issues like ambiguity and complexity. Proper understanding and application of these inheritance types can lead to robust and maintainable object-oriented systems Types of Inheritance in C++